So finden Sie Vokale, Konsonanten, Ziffern und Sonderzeichen in einer Zeichenfolge
Ein String ist eine Folge von Zeichen. Diese Zeichen können Vokale, Konsonanten, Ziffern oder beliebige Sonderzeichen sein. In diesem Artikel erfahren Sie, wie Sie die Gesamtzahl der Vokale, Konsonanten, Ziffern und Sonderzeichen in einer bestimmten Zeichenfolge ermitteln.
Beispiele zum Verständnis des Problems
Beispiel 1 : Die angegebene Zeichenfolge sei "Welcome 2 #MUO".
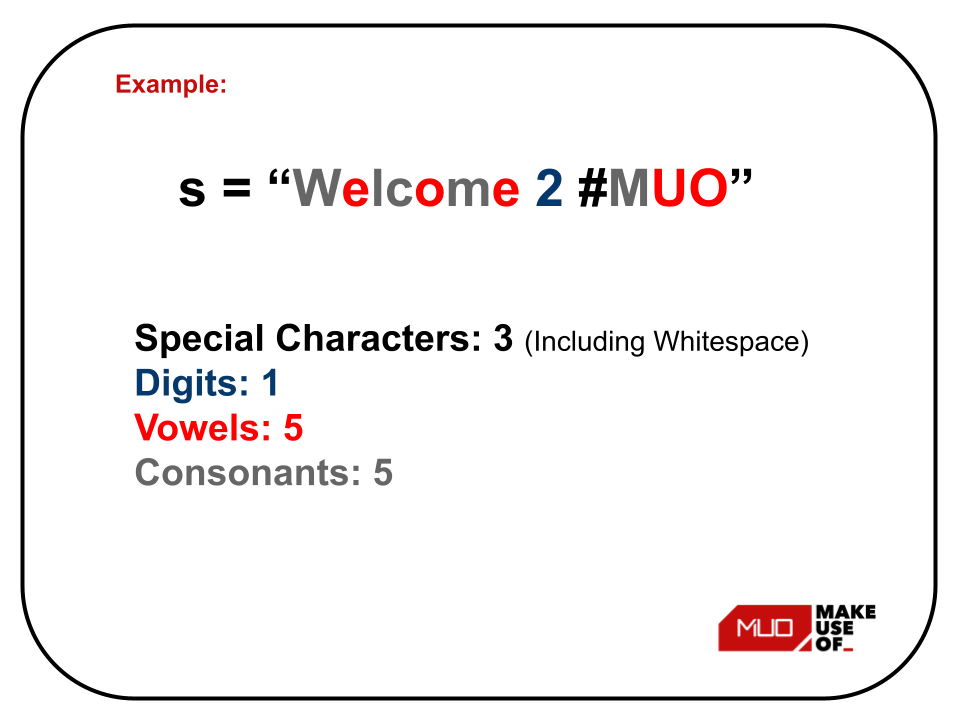
s = "Willkommen 2 #MUO"
Es gibt 5 Vokale in der gegebenen Zeichenfolge: e , o , e , U und O .
Es gibt 5 Konsonanten in der gegebenen Zeichenfolge: W , l , c , m und M .
Es gibt 1 Ziffer in der angegebenen Zeichenfolge: 2 .
Es gibt 3 Sonderzeichen in der angegebenen Zeichenfolge: # und zwei Leerzeichen.
Beispiel 2: Der angegebene String sei "This is @ inpuT String 2".
s = "Dies ist @ Eingabezeichenfolge 2"
Es gibt 5 Vokale in der angegebenen Zeichenfolge: i , I , I , u und i .
Es gibt 12 Konsonanten in der gegebenen Zeichenfolge: T , h , s , s , n , p , T , S , t , r , n und g .
Es gibt 1 Ziffer in der angegebenen Zeichenfolge: 2 .
Die angegebene Zeichenfolge enthält 6 Sonderzeichen: @ und fünf Leerzeichen.
Hinweis: Leerzeichen werden in der Zeichenfolge als Sonderzeichen behandelt.
Ansatz zum Zählen von Vokalen, Konsonanten, Ziffern und Sonderzeichen in einer Zeichenfolge
Sie können die Gesamtzahl der Vokale, Konsonanten, Ziffern und Sonderzeichen in einer Zeichenfolge wie folgt ermitteln:
- Initialisieren Sie Variablen, um die Gesamtzahl der Vokale, Konsonanten, Ziffern und Sonderzeichen zu zählen.
- Durchlaufe die angegebene Zeichenfolge zeichenweise.
- Prüfen Sie, ob das Zeichen zur Alphabetfamilie, Ziffernfamilie oder Sonderzeichenfamilie gehört.
- Wenn das Zeichen zur Alphabetfamilie gehört, konvertieren Sie es zuerst in Kleinbuchstaben und prüfen Sie dann, ob es sich um einen Vokal oder einen Konsonanten handelt.
- Wenn das Zeichen ein Vokal ist, erhöhen Sie den Wert der Variablen, die die Gesamtzahl der Vokale in einer Zeichenfolge speichert.
- Andernfalls, wenn das Zeichen ein Konsonant ist, erhöhen Sie den Wert der Variablen, die die Gesamtzahl der Konsonanten in einer Zeichenfolge speichert.
- Wenn das Zeichen zur Ziffernfamilie gehört, inkrementieren Sie den Wert der Variablen, der die Gesamtzahl der Ziffern in einer Zeichenfolge speichert.
- Wenn das Zeichen zur Sonderzeichenfamilie gehört, erhöhen Sie den Wert der Variablen, der die Gesamtzahl der Sonderzeichen in einer Zeichenfolge speichert.
C++-Programm zum Zählen von Vokalen, Konsonanten, Ziffern und Sonderzeichen in einem String
Unten ist das C++-Programm zum Zählen von Vokalen, Konsonanten, Ziffern und Sonderzeichen in einer Zeichenfolge:
#include <iostream>
using namespace std;
void countCharactersCategory(string s)
{
int totalSpecialCharacters = 0, totalDigits = 0, totalVowels = 0, totalConsonants = 0;
for (int i = 0; i < s.length(); i++)
{
char c = s[i];
// Alphabets family
if ( (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z') )
{
// Converting character to lower case
c = tolower(c);
// Vowels
if (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u')
{
totalVowels++;
}
// Consonants
else
{
totalConsonants++;
}
}
// Digits family
else if (c >= '0' && c <= '9')
{
totalDigits++;
}
// Special characters family
else
{
totalSpecialCharacters++;
}
}
cout << "Total no. of vowels in the given string: " << totalVowels << endl;
cout << "Total no. of consonants in the given string: " << totalConsonants << endl;
cout << "Total no. of digits in the given string: " << totalDigits << endl;
cout << "Total no. of special characters in the given string: " << totalSpecialCharacters << endl;
}
// Driver code
int main()
{
// Test case: 1
string s1 = "Welcome 2 #MUO";
cout << "Input string: " << s1 << endl;
countCharactersCategory(s1);
// Test case: 2
string s2 = "This Is @ InpuT String 2";
cout << "Input string: " << s2 << endl;
countCharactersCategory(s2);
return 0;
}
Ausgabe:
Input string: Welcome 2 #MUO
Total no. of vowels in the given string: 5
Total no. of consonants in the given string: 5
Total no. of digits in the given string: 1
Total no. of special characters in the given string: 3
Input string: This Is @ InpuT String 2
Total no. of vowels in the given string: 5
Total no. of consonants in the given string: 12
Total no. of digits in the given string: 1
Total no. of special characters in the given string: 6
Python-Programm zum Zählen von Vokalen, Konsonanten, Ziffern und Sonderzeichen in einer Zeichenfolge
Unten ist das Python-Programm zum Zählen von Vokalen, Konsonanten, Ziffern und Sonderzeichen in einer Zeichenfolge:
def countCharactersCategory(s):
totalSpecialCharacters = 0
totalDigits = 0
totalVowels = 0
totalConsonants = 0
for i in range(0, len(s)):
c = s[i]
# Alphabets family
if ( (c >= 'a' and c = 'A' and c = '0' and c <= '9'):
totalDigits += 1
# Special characters family
else:
totalSpecialCharacters += 1
print("Total no. of vowels in the given string: ", totalVowels)
print("Total no. of consonants in the given string: ", totalConsonants)
print("Total no. of digits in the given string: ", totalDigits)
print("Total no. of special characters in the given string: ", totalSpecialCharacters)
# Driver code
# Test case: 1
s1 = "Welcome 2 #MUO"
print("Input string: ", s1)
countCharactersCategory(s1)
# Test case: 2
s2 = "This Is @ InpuT String 2"
print("Input string: ", s2)
countCharactersCategory(s2)
Ausgabe:
Input string: Welcome 2 #MUO
Total no. of vowels in the given string: 5
Total no. of consonants in the given string: 5
Total no. of digits in the given string: 1
Total no. of special characters in the given string: 3
Input string: This Is @ InpuT String 2
Total no. of vowels in the given string: 5
Total no. of consonants in the given string: 12
Total no. of digits in the given string: 1
Total no. of special characters in the given string: 6
C Programm zum Zählen von Vokalen, Konsonanten, Ziffern und Sonderzeichen in einer Zeichenfolge
Unten ist das C-Programm zum Zählen von Vokalen, Konsonanten, Ziffern und Sonderzeichen in einer Zeichenfolge:
#include <stdio.h>
#include <ctype.h>
#include <string.h>
void countCharactersCategory(char s[])
{
int totalSpecialCharacters = 0, totalDigits = 0, totalVowels = 0, totalConsonants = 0;
for (int i = 0; i < strlen(s); i++)
{
char c = s[i];
// Alphabets family
if ( (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z') )
{
// Converting character to lower case
c = tolower(c);
// Vowels
if (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u')
{
totalVowels++;
}
// Consonants
else
{
totalConsonants++;
}
}
// Digits family
else if (c >= '0' && c <= '9')
{
totalDigits++;
}
// Special characters family
else
{
totalSpecialCharacters++;
}
}
printf("Total no. of vowels in the given string: %d n",totalVowels);
printf("Total no. of consonants in the given string: %d n",totalConsonants);
printf("Total no. of digits in the given string: %d n",totalDigits);
printf("Total no. of special characters in the given string: %d n",totalSpecialCharacters);
}
// Driver code
int main()
{
// Test case: 1
char s1[] = "Welcome 2 #MUO";
printf("Input string: %s
",s1);
countCharactersCategory(s1);
// Test case: 2
char s2[] = "This Is @ InpuT String 2";
printf("Input string: %s
",s2);
countCharactersCategory(s2);
return 0;
}
Ausgabe:
Input string: Welcome 2 #MUO
Total no. of vowels in the given string: 5
Total no. of consonants in the given string: 5
Total no. of digits in the given string: 1
Total no. of special characters in the given string: 3
Input string: This Is @ InpuT String 2
Total no. of vowels in the given string: 5
Total no. of consonants in the given string: 12
Total no. of digits in the given string: 1
Total no. of special characters in the given string: 6
JavaScript-Programm zum Zählen von Vokalen, Konsonanten, Ziffern und Sonderzeichen in einer Zeichenfolge
Unten ist das JavaScript-Programm zum Zählen von Vokalen, Konsonanten, Ziffern und Sonderzeichen in einer Zeichenfolge:
<script>
function countCharactersCategory(s) {
var totalSpecialCharacters = 0, totalDigits = 0, totalVowels = 0, totalConsonants = 0;
for (var i = 0; i < s.length; i++) {
var c = s[i];
// Alphabets family
if ( (c >= "a" && c <= "z") || (c >= "A" && c <= "Z") ) {
// Converting character to lower case
c = c.toLowerCase();
// Vowels
if (c == "a" || c == "e" || c == "i" || c == "o" || c == "u") {
totalVowels++;
}
// Consonants
else {
totalConsonants++;
}
}
// Digits family
else if (c >= "0" && c <= "9") {
totalDigits++;
}
// Special characters family
else {
totalSpecialCharacters++;
}
}
document.write("Total no. of vowels in the given string: " + totalVowels + "<br>");
document.write("Total no. of consonants in the given string: " + totalConsonants + "<br>");
document.write("Total no. of digits in the given string: " + totalDigits + "<br>");
document.write("Total no. of special characters in the given string: " + totalSpecialCharacters + "<br>");
}
// Test case: 1
var s1 = "Welcome 2 #MUO";
document.write("Input string: " + s1 + "<br>");
countCharactersCategory(s1);
// Test case: 2
var s2 = "This Is @ InpuT String 2";
document.write("Input string: " + s2 + "<br>");
countCharactersCategory(s2);
</script>
Ausgabe:
Input string: Welcome 2 #MUO
Total no. of vowels in the given string: 5
Total no. of consonants in the given string: 5
Total no. of digits in the given string: 1
Total no. of special characters in the given string: 3
Input string: This Is @ InpuT String 2
Total no. of vowels in the given string: 5
Total no. of consonants in the given string: 12
Total no. of digits in the given string: 1
Total no. of special characters in the given string: 6
Wenn Sie sich den vollständigen Quellcode, der in diesem Artikel verwendet wird, ansehen möchten, finden Sie hier das GitHub-Repository .
Üben Sie String-Probleme für Ihre Interviews
String-Probleme sind eine der am häufigsten gestellten Fragen bei Coding-Wettbewerben und -Interviews. Verstehen Sie die Grundlagen der Saiten und üben Sie berühmte Probleme, um ein besserer Ingenieur zu werden.
Das Entfernen doppelter Zeichen aus einem String, das Finden des maximal vorkommenden Zeichens in einem String und das Prüfen, ob ein String ein Palindrom ist, sind einige der bekanntesten String-Probleme.
Warum nicht auch diese Probleme ausprobieren?