So addieren und subtrahieren Sie zwei Matrizen in C++, Python und JavaScript
Eine Matrix ist ein rechteckiges Array von Zahlen, Symbolen oder Ausdrücken, die in Zeilen und Spalten angeordnet sind. Dieses rechteckige Zahlenraster wird häufig in Mathematik, Elektrotechnik und Informatik verwendet. Matrizen wurden ursprünglich erstellt, um das System linearer Gleichungen zu beschreiben.
Heute werden Matrizen häufig in der Bildverarbeitung, Genanalyse, Big Data und Programmierung verwendet. Die Addition und Subtraktion von Matrizen sind die beiden häufigsten Matrizenoperationen. In diesem Artikel erfahren Sie, wie Sie zwei Matrizen addieren und subtrahieren.
Regeln für die Matrixaddition
Befolgen Sie diese Regeln, um zwei Matrizen hinzuzufügen:
- Zwei Matrizen können nur hinzugefügt werden, wenn sie von derselben Reihenfolge sind.
- Wenn die beiden Matrizen dieselbe Ordnung haben, addieren Sie die entsprechenden Elemente der beiden Matrizen, dh addieren Sie die Elemente, die die gleichen Positionen haben.
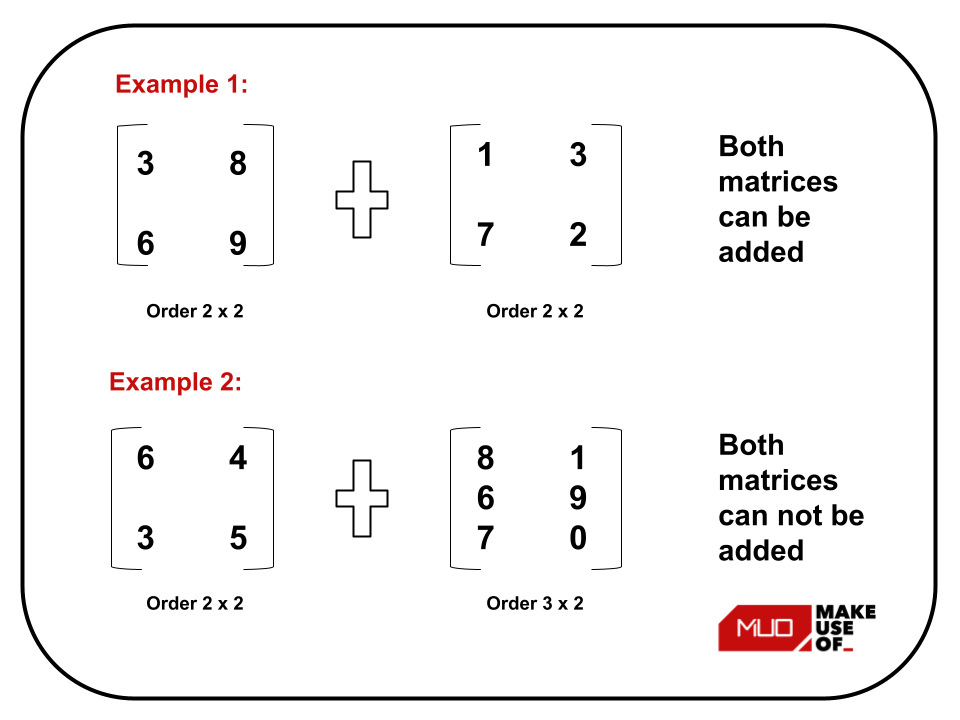
In Beispiel 1 können die Matrizen hinzugefügt werden, da sie die gleiche Reihenfolge haben. In Beispiel 2 können die Matrizen nicht hinzugefügt werden, da sie nicht dieselbe Reihenfolge haben.
C++-Programm zum Hinzufügen von zwei Matrizen
Unten ist das C++-Programm zum Hinzufügen von zwei Matrizen:
// C++ program for addition of two matrices
#include <bits/stdc++.h>
using namespace std;
// The order of the matrix is 3 x 3
#define size1 3
#define size2 3
// Function to add matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
void addMatrices(int mat1[][size2], int mat2[][size2], int result[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
result[i][j] = mat1[i][j] + mat2[i][j];
}
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {9, 8, 7},
{6, 8, 0},
{5, 9, 2} };
// 2nd Matrix
int mat2[size1][size2] = { {4, 7, 6},
{8, 8, 2},
{7, 3, 5} };
// Matrix to store the result
int result[size1][size2];
// Calling the addMatrices() function
addMatrices(mat1, mat2, result);
cout << "mat1 + mat2 = " << endl;
// Printing the sum of the 2 matrices
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
cout << result[i][j] << " ";
}
cout << endl;
}
return 0;
}
Ausgabe:
mat1 + mat2 =
13 15 13
14 16 2
12 12 7
Python-Programm zum Hinzufügen von zwei Matrizen
Unten ist das Python-Programm zum Hinzufügen von zwei Matrizen:
# Python program for addition of two matrices
# The order of the matrix is 3 x 3
size1 = 3
size2 = 3
# Function to add matrices mat1[][] & mat2[][],
# and store the result in matrix result[][]
def addMatrices(mat1,mat2,result):
for i in range(size1):
for j in range(size2):
result[i][j] = mat1[i][j] + mat2[i][j]
# driver code
# 1st Matrix
mat1 = [ [9, 8, 7],
[6, 8, 0],
[5, 9, 2] ]
# 2nd Matrix
mat2 = [ [4, 7, 6],
[8, 8, 2],
[7, 3, 5] ]
# Matrix to store the result
result = mat1[:][:]
# Calling the addMatrices function
addMatrices(mat1, mat2, result)
# Printing the sum of the 2 matrices
print("mat1 + mat2 = ")
for i in range(size1):
for j in range(size2):
print(result[i][j], " ", end='')
print()
Ausgabe:
mat1 + mat2 =
13 15 13
14 16 2
12 12 7
C-Programm zum Hinzufügen von zwei Matrizen
Unten ist das C-Programm zum Hinzufügen von zwei Matrizen:
// C program for addition of two matrices
#include <stdio.h>
// The order of the matrix is 3 x 3
#define size1 3
#define size2 3
// Function to add matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
void addMatrices(int mat1[][size2], int mat2[][size2], int result[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
result[i][j] = mat1[i][j] + mat2[i][j];
}
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {9, 8, 7},
{6, 8, 0},
{5, 9, 2} };
// 2nd Matrix
int mat2[size1][size2] = { {4, 7, 6},
{8, 8, 2},
{7, 3, 5} };
// Matrix to store the result
int result[size1][size2];
// Calling the addMatrices function
addMatrices(mat1, mat2, result);
printf("mat1 + mat2 = n");
// Printing the sum of the 2 matrices
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
printf("%d ", result[i][j]);
}
printf("n");
}
return 0;
}
Ausgabe:
mat1 + mat2 =
13 15 13
14 16 2
12 12 7
JavaScript-Programm zum Hinzufügen von zwei Matrizen
Unten ist das JavaScript-Programm zum Hinzufügen von zwei Matrizen:
<script>
// JavaScript program for addition of two matrices
// The order of the matrix is 3 x 3
let size1 = 3;
let size2 = 3;
// Function to add matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
function addMatrices(mat1, mat2, result) {
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
result[i][j] = mat1[i][j] + mat2[i][j];
}
}
}
// Driver code
// 1st Matrix
mat1 = [ [9, 8, 7],
[6, 8, 0],
[5, 9, 2] ]
// 2nd Matrix
mat2 = [ [4, 7, 6],
[8, 8, 2],
[7, 3, 5] ]
// Matrix to store the result
let result = new Array(size1);
for (let k = 0; k < size1; k++) {
result[k] = new Array(size2);
}
// Calling the addMatrices function
addMatrices(mat1, mat2, result);
document.write("mat1 + mat2 = <br>")
// Printing the sum of the 2 matrices
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
document.write(result[i][j] + " ");
}
document.write("<br>");
}
</script>
Ausgabe:
mat1 + mat2 =
13 15 13
14 16 2
12 12 7
Regeln für die Matrixsubtraktion
Befolgen Sie diese Regeln, um zwei Matrizen zu subtrahieren:
- Zwei Matrizen können nur subtrahiert werden, wenn sie dieselbe Ordnung haben.
- Wenn die beiden Matrizen die gleiche Ordnung haben, subtrahieren Sie die entsprechenden Elemente der beiden Matrizen, dh subtrahieren Sie die Elemente, die die gleichen Positionen haben.
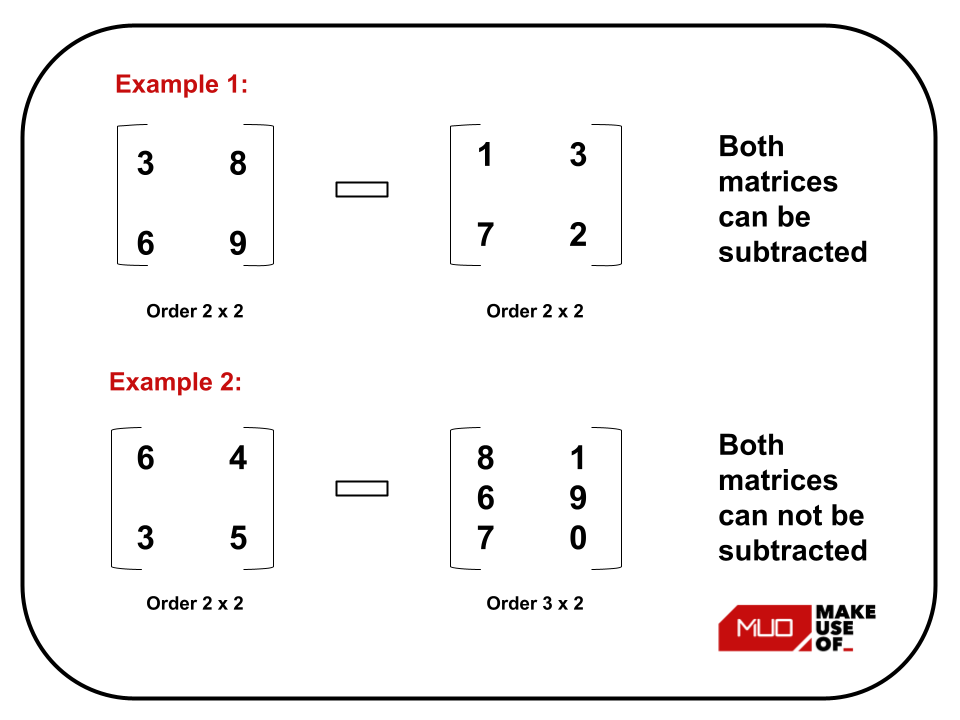
In Beispiel 1 können die Matrizen subtrahiert werden, da sie die gleiche Reihenfolge haben. In Beispiel 2 können die Matrizen nicht subtrahiert werden, da sie nicht dieselbe Reihenfolge haben.
C++-Programm zum Subtrahieren von zwei Matrizen
Unten ist das C++-Programm zum Subtrahieren von zwei Matrizen:
// C++ program for subtraction of two matrices
#include <bits/stdc++.h>
using namespace std;
// The order of the matrix is 3 x 3
#define size1 3
#define size2 3
// Function to subtract matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
void subtractMatrices(int mat1[][size2], int mat2[][size2], int result[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
result[i][j] = mat1[i][j] - mat2[i][j];
}
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {9, 8, 7},
{6, 8, 0},
{5, 9, 2} };
// 2nd Matrix
int mat2[size1][size2] = { {4, 7, 6},
{8, 8, 2},
{7, 3, 5} };
// Matrix to store the result
int result[size1][size2];
// Calling the subtractMatrices() function
subtractMatrices(mat1, mat2, result);
cout << "mat1 - mat2 = " << endl;
// Printing the difference of the 2 matrices (mat1 - mat2)
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
cout << result[i][j] << " ";
}
cout << endl;
}
return 0;
}
Ausgabe:
mat1 - mat2 =
5 1 1
-2 0 -2
-2 6 -3
Python-Programm zum Subtrahieren von zwei Matrizen
Unten ist das Python-Programm zum Subtrahieren von zwei Matrizen:
# Python program for subtraction of two matrices
# The order of the matrix is 3 x 3
size1 = 3
size2 = 3
# Function to subtract matrices mat1[][] & mat2[][],
# and store the result in matrix result[][]
def subtractMatrices(mat1,mat2,result):
for i in range(size1):
for j in range(size2):
result[i][j] = mat1[i][j] - mat2[i][j]
# driver code
# 1st Matrix
mat1 = [ [9, 8, 7],
[6, 8, 0],
[5, 9, 2] ]
# 2nd Matrix
mat2 = [ [4, 7, 6],
[8, 8, 2],
[7, 3, 5] ]
# Matrix to store the result
result = mat1[:][:]
# Calling the subtractMatrices function
subtractMatrices(mat1, mat2, result)
# Printing the difference of the 2 matrices (mat1 - mat2)
print("mat1 - mat2 = ")
for i in range(size1):
for j in range(size2):
print(result[i][j], " ", end='')
print()
Ausgabe:
mat1 - mat2 =
5 1 1
-2 0 -2
-2 6 -3
C-Programm zum Subtrahieren von zwei Matrizen
Unten ist das C-Programm zum Subtrahieren von zwei Matrizen:
// C program for subtraction of two matrices
#include <stdio.h>
// The order of the matrix is 3 x 3
#define size1 3
#define size2 3
// Function to subtract matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
void subtractMatrices(int mat1[][size2], int mat2[][size2], int result[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
result[i][j] = mat1[i][j] - mat2[i][j];
}
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {9, 8, 7},
{6, 8, 0},
{5, 9, 2} };
// 2nd Matrix
int mat2[size1][size2] = { {4, 7, 6},
{8, 8, 2},
{7, 3, 5} };
// Matrix to store the result
int result[size1][size2];
// Calling the subtractMatrices() function
subtractMatrices(mat1, mat2, result);
printf("mat1 - mat2 = n");
// Printing the difference of the 2 matrices (mat1 - mat2)
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
printf("%d ", result[i][j]);
}
printf("n");
}
return 0;
}
Ausgabe:
mat1 - mat2 =
5 1 1
-2 0 -2
-2 6 -3
JavaScript-Programm zum Subtrahieren von zwei Matrizen
Unten ist das JavaScript-Programm zum Subtrahieren von zwei Matrizen:
<script>
// JavaScript program for subtraction of two matrices
// The order of the matrix is 3 x 3
let size1 = 3;
let size2 = 3;
// Function to subtract matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
function subtractMatrices(mat1, mat2, result) {
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
result[i][j] = mat1[i][j] - mat2[i][j];
}
}
}
// Driver code
// 1st Matrix
mat1 = [ [9, 8, 7],
[6, 8, 0],
[5, 9, 2] ]
// 2nd Matrix
mat2 = [ [4, 7, 6],
[8, 8, 2],
[7, 3, 5]]
// Matrix to store the result
let result = new Array(size1);
for (let k = 0; k < size1; k++) {
result[k] = new Array(size2);
}
// Calling the subtractMatrices function
subtractMatrices(mat1, mat2, result);
document.write("mat1 - mat2 = <br>")
// Printing the difference of the 2 matrices (mat1 - mat2)
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
document.write(result[i][j] + " ");
}
document.write("<br>");
}
</script>
Ausgabe:
mat1 - mat2 =
5 1 1
-2 0 -2
-2 6 -3
Wenn Sie sich den vollständigen Quellcode, der in diesem Artikel verwendet wird, ansehen möchten, finden Sie hier das GitHub-Repository .
Erhöhen Sie Ihre Programmierfähigkeit
Sie können Ihre Programmierfähigkeiten verbessern, indem Sie eine Vielzahl von Programmierproblemen üben. Die Lösung dieser Programmierprobleme hilft Ihnen, grundlegende Programmierprinzipien zu entwickeln. Diese sind ein Muss, wenn Sie ein effizienter Programmierer werden wollen.