So überprüfen Sie, ob zwei Matrizen mit der Programmierung identisch sind
Zwei Matrizen heißen identisch, wenn beide die gleiche Anzahl von Zeilen, Spalten und die gleichen entsprechenden Elemente haben. In diesem Artikel erfahren Sie, wie Sie mit Python, C++, JavaScript und C überprüfen können, ob zwei Matrizen identisch sind.
Problemstellung
Sie erhalten zwei Matrizen mat1[][] und mat2[][] . Sie müssen überprüfen, ob die beiden Matrizen identisch sind. Wenn die beiden Matrizen identisch sind, geben Sie "Ja, die Matrizen sind identisch" aus. Und wenn die beiden Matrizen nicht identisch sind, geben Sie "Nein, die Matrizen sind nicht identisch" aus.
Beispiele :
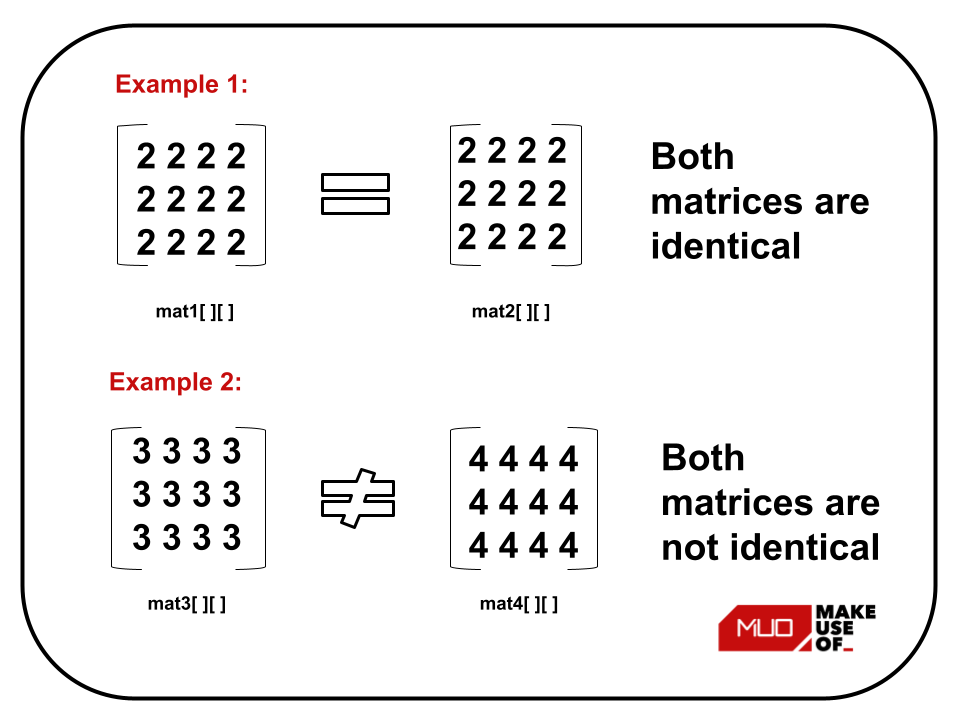
Bedingung, dass zwei Matrizen identisch sind
Zwei Matrizen heißen genau dann identisch, wenn sie die folgenden Bedingungen erfüllen:
- Beide Matrizen haben die gleiche Anzahl von Zeilen und Spalten.
- Beide Matrizen haben die gleichen entsprechenden Elemente.
Ansatz, um zu überprüfen, ob die beiden gegebenen Matrizen identisch sind
Sie können dem folgenden Ansatz folgen, um zu überprüfen, ob die beiden angegebenen Matrizen identisch sind oder nicht:
- Führen Sie eine verschachtelte Schleife aus, um jedes Element beider Matrizen zu durchlaufen.
- Wenn eines der entsprechenden Elemente der beiden Matrizen nicht gleich ist, wird false zurückgegeben.
- Und wenn bis zum Ende der Schleife keine entsprechenden Elemente gefunden werden, die unähnlich sind, geben Sie true zurück.
C++-Programm, um zu überprüfen, ob die beiden gegebenen Matrizen identisch sind
Unten ist das C++-Programm, um zu überprüfen, ob die beiden angegebenen Matrizen identisch sind oder nicht:
// C++ program to check if two matrices are identical
#include <bits/stdc++.h>
using namespace std;
// The order of the matrix is 3 x 4
#define size1 3
#define size2 4
// Function to check if two matrices are identical
bool isIdentical(int mat1[][size2], int mat2[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
if (mat1[i][j] != mat2[i][j])
{
return false;
}
}
}
return true;
}
// Function to print a matrix
void printMatrix(int mat[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
cout << mat[i][j] << " ";
}
cout << endl;
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
cout << "Matrix 1:" << endl;
printMatrix(mat1);
// 2nd Matrix
int mat2[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
cout << "Matrix 2:" << endl;
printMatrix(mat2);
if(isIdentical(mat1, mat2))
{
cout << "Yes, the matrices are identical" << endl;
}
else
{
cout << "No, the matrices are not identical" << endl;
}
// 3rd Matrix
int mat3[size1][size2] = { {3, 3, 3, 3},
{3, 3, 3, 3},
{3, 3, 3, 3} };
cout << "Matrix 3:" << endl;
printMatrix(mat3);
// 4th Matrix
int mat4[size1][size2] = { {4, 4, 4, 4},
{4, 4, 4, 4},
{4, 4, 4, 4} };
cout << "Matrix 4:" << endl;
printMatrix(mat4);
if(isIdentical(mat3, mat4))
{
cout << "Yes, the matrices are identical" << endl;
}
else
{
cout << "No, the matrices are not identical" << endl;
}
return 0;
}
Ausgabe:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
Python-Programm, um zu überprüfen, ob die beiden gegebenen Matrizen identisch sind
Unten ist das Python-Programm, um zu überprüfen, ob die beiden angegebenen Matrizen identisch sind oder nicht:
# Python program to check if two matrices are identical
# The order of the matrix is 3 x 4
size1 = 3
size2 = 4
# Function to check if two matrices are identical
def isIdentical(mat1, mat2):
for i in range(size1):
for j in range(size2):
if (mat1[i][j] != mat2[i][j]):
return False
return True
# Function to print a matrix
def printMatrix(mat):
for i in range(size1):
for j in range(size2):
print(mat[i][j], end=' ')
print()
# Driver code
# 1st Matrix
mat1 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ]
print("Matrix 1:")
printMatrix(mat1)
# 2nd Matrix
mat2 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ]
print("Matrix 2:")
printMatrix(mat2)
if (isIdentical(mat1, mat2)):
print("Yes, the matrices are identical")
else:
print("No, the matrices are not identical")
# 3rd Matrix
mat3 = [ [3, 3, 3, 3],
[3, 3, 3, 3],
[3, 3, 3, 3] ]
print("Matrix 3:")
printMatrix(mat3)
# 4th Matrix
mat4 = [ [4, 4, 4, 4],
[4, 4, 4, 4],
[4, 4, 4, 4] ]
print("Matrix 4:")
printMatrix(mat4)
if (isIdentical(mat3, mat4)):
print("Yes, the matrices are identical")
else:
print("No, the matrices are not identical")
Ausgabe:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
JavaScript-Programm, um zu überprüfen, ob die beiden gegebenen Matrizen identisch sind
Unten ist das JavaScript-Programm, um zu überprüfen, ob die beiden angegebenen Matrizen identisch sind oder nicht:
// JavaScript program to check if two matrices are identical
// The order of the matrix is 3 x 4
var size1 = 3;
var size2 = 4;
// Function to check if two matrices are identical
function isIdentical(mat1, mat2) {
for (let i = 0; i < size1; i++)
{
for (let j = 0; j < size2; j++)
{
if (mat1[i][j] != mat2[i][j])
{
return false;
}
}
}
return true;
}
// Function to print a matrix
function printMatrix(mat) {
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
document.write(mat[i][j] + " ");
}
document.write("<br>");
}
}
// Driver code
// 1st Matrix
var mat1 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ];
document.write("Matrix 1:" + "<br>");
printMatrix(mat1);
// 2nd Matrix
var mat2 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ];
document.write("Matrix 2:" + "<br>");
printMatrix(mat2);
if (isIdentical(mat1, mat2)) {
document.write("Yes, the matrices are identical" + "<br>");
} else{
document.write("No, the matrices are not identical" + "<br>");
}
// 3rd Matrix
var mat3 = [ [3, 3, 3, 3],
[3, 3, 3, 3],
[3, 3, 3, 3] ];
document.write("Matrix 3:" + "<br>");
printMatrix(mat3);
// 4th Matrix
var mat4 = [ [4, 4, 4, 4],
[4, 4, 4, 4],
[4, 4, 4, 4] ];
document.write("Matrix 4:" + "<br>");
printMatrix(mat4);
if (isIdentical(mat3, mat4)) {
document.write("Yes, the matrices are identical" + "<br>");
} else{
document.write("No, the matrices are not identical" + "<br>");
}
Ausgabe:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
C Programm zur Überprüfung, ob die beiden gegebenen Matrizen identisch sind
Unten ist das C-Programm, um zu überprüfen, ob die beiden angegebenen Matrizen identisch sind oder nicht:
// C program to check if two matrices are identical
#include <stdio.h>
#include <stdbool.h>
// The order of the matrix is 3 x 4
#define size1 3
#define size2 4
// Function to check if two matrices are identical
bool isIdentical(int mat1[][size2], int mat2[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
if (mat1[i][j] != mat2[i][j])
{
return false;
}
}
}
return true;
}
// Function to print a matrix
void printMatrix(int mat[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
printf("%d ", mat[i][j]);
}
printf("n");
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
printf("Matrix 1:n");
printMatrix(mat1);
// 2nd Matrix
int mat2[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
printf("Matrix 2:n");
printMatrix(mat2);
if(isIdentical(mat1, mat2))
{
printf("Yes, the matrices are identical n");
}
else
{
printf("No, the matrices are not identical n");
}
// 3rd Matrix
int mat3[size1][size2] = { {3, 3, 3, 3},
{3, 3, 3, 3},
{3, 3, 3, 3} };
printf("Matrix 3: n");
printMatrix(mat3);
// 4th Matrix
int mat4[size1][size2] = { {4, 4, 4, 4},
{4, 4, 4, 4},
{4, 4, 4, 4} };
printf("Matrix 4: n");
printMatrix(mat4);
if(isIdentical(mat3, mat4))
{
printf("Yes, the matrices are identical n");
}
else
{
printf("No, the matrices are not identical n");
}
return 0;
}
Ausgabe:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
Lernen Sie eine neue Programmiersprache
Die Informatik expandiert sehr schnell und der Wettbewerb in diesem Bereich ist intensiver denn je. Sie müssen sich mit den neuesten Kenntnissen und Programmiersprachen auf dem Laufenden halten. Egal ob Anfänger oder erfahrener Programmierer, in jedem Fall sollten Sie einige der Programmiersprachen nach Branchenanforderungen erlernen.